Vue Dynamic Componentsの使い方
Vue 3 Nuxt 3 Dynamic components are useful when you are unsure of what content you want to display or in what order. This article shows how to use dynamic components in Vue 3. The example uses Nuxt 3.
by JanitorSep 10, 2023
Dynamic Componentsの使い方の記事です。表示させたいコンテンツが、何なのか、またどのような順番でくるのか不明な時などに使うと便利です。
Dynamic Componentsの使い方の記事です。表示させたいコンテンツが、何なのか、またどのような順番でくるのか不明な時などに使うと便利です。
Jump Links
1. pagesフォルダにcomponentStudyフォルダを作成
2. componentを作成
3. 動作確認
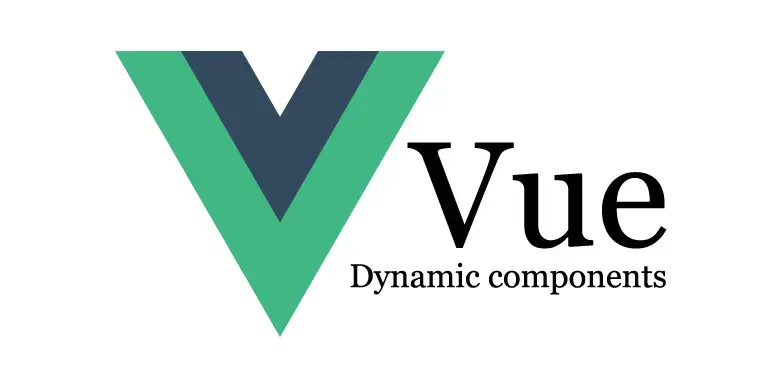
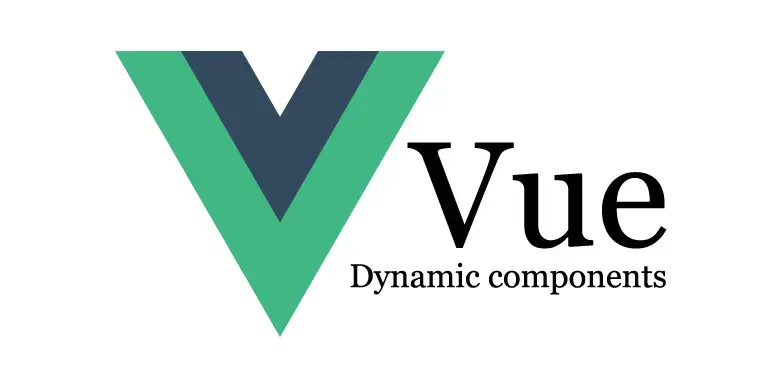
1. pagesフォルダにcomponentStudyフォルダを作成
1. pagesフォルダにcomponentStudyフォルダを作成
フォルダ名は何でも構いません。今回は「componentStudy」としています。直下にindex.vueを置きます。
フォルダ名は何でも構いません。今回は「componentStudy」としています。直下にindex.vueを置きます。
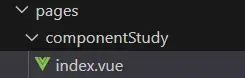
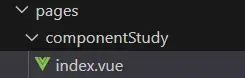
index.vueの内容は下記のようにして確認しています。やっていることは、表示データを準備し、その表示データのプロパティ「kind」によってvueを設定し、componentタグのis属性に設定したvueを読み込ませています。
index.vueの内容は下記のようにして確認しています。やっていることは、表示データを準備し、その表示データのプロパティ「kind」によってvueを設定し、componentタグのis属性に設定したvueを読み込ませています。
<script setup>
import fontSizeLargeVue from '@/components/fontSizeLarge.vue'
import fontSizeSmallVue from '@/components/fontSizeSmall.vue'
import imageVue from '@/components/image.vue'
// API等で表示データを取得
var contents = [
{ kind: "fontSizeLarge", arg: "This text will be displayed in large letters." },
{ kind: "fontSizeSmall", arg: "This text will be displayed in small letters." },
{ kind: "img", arg: "https://comytom.com/C250.webp" },
];
// どのvueを使うかを決定
for( var i = 0; i < contents.length; i++ ){
if( contents[ i ].kind == "fontSizeLarge" ) contents[ i ].vue = fontSizeLargeVue;
else if( contents[ i ].kind == "fontSizeSmall" ) contents[ i ].vue = fontSizeSmallVue;
else if( contents[ i ].kind == "img" ) contents[ i ].vue = imageVue;
}
</script>
<template>
<VContainer fluid class="h-100">
<component v-for="(content, i) in contents" :key="i" :is="{...content.vue}" :arg="content.arg"></component>
</VContainer>
</template>
<script setup>
import fontSizeLargeVue from '@/components/fontSizeLarge.vue'
import fontSizeSmallVue from '@/components/fontSizeSmall.vue'
import imageVue from '@/components/image.vue'
// API等で表示データを取得
var contents = [
{ kind: "fontSizeLarge", arg: "This text will be displayed in large letters." },
{ kind: "fontSizeSmall", arg: "This text will be displayed in small letters." },
{ kind: "img", arg: "https://comytom.com/C250.webp" },
];
// どのvueを使うかを決定
for( var i = 0; i < contents.length; i++ ){
if( contents[ i ].kind == "fontSizeLarge" ) contents[ i ].vue = fontSizeLargeVue;
else if( contents[ i ].kind == "fontSizeSmall" ) contents[ i ].vue = fontSizeSmallVue;
else if( contents[ i ].kind == "img" ) contents[ i ].vue = imageVue;
}
</script>
<template>
<VContainer fluid class="h-100">
<component v-for="(content, i) in contents" :key="i" :is="{...content.vue}" :arg="content.arg"></component>
</VContainer>
</template>
2. componentを作成
2. componentを作成
プロジェクトフォルダのcomponentsフォルダ(無い場合は作成)内に、上のスクリプトでimportしているコンポーネント3つ(fontSizeLarge.vue、fontSizeSmall.vue、image.vue)を作成します。
プロジェクトフォルダのcomponentsフォルダ(無い場合は作成)内に、上のスクリプトでimportしているコンポーネント3つ(fontSizeLarge.vue、fontSizeSmall.vue、image.vue)を作成します。
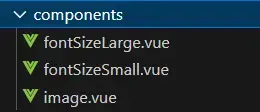
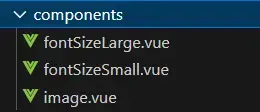
それぞれ下記のようにして、動的コンポーネントの動作を確認できます。
それぞれ下記のようにして、動的コンポーネントの動作を確認できます。
fontSizeLarge.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeLargeStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeLargeStyle{
font-size: 1.5rem;
}
</style>
fontSizeLarge.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeLargeStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeLargeStyle{
font-size: 1.5rem;
}
</style>
fontSizeSmall.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeSmallStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeSmallStyle{
font-size: 0.8rem;
}
</style>
fontSizeSmall.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeSmallStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeSmallStyle{
font-size: 0.8rem;
}
</style>
image.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<img :src="arg"
class="imageStyle">
</template>
<style>
.imageStyle{
width: 250px;
height: 250px;
}
</style>
image.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<img :src="arg"
class="imageStyle">
</template>
<style>
.imageStyle{
width: 250px;
height: 250px;
}
</style>
参考にさせて頂いたウェブサイトURL
https://blog.logrocket.com/making-components-dynamic-vue-3/
参考にさせて頂いたウェブサイトURL
https://blog.logrocket.com/making-components-dynamic-vue-3/
3. 動作確認
3. 動作確認
yarn devしてcomponentStudyにアクセスすると下の画像のような表示になります。
yarn devしてcomponentStudyにアクセスすると下の画像のような表示になります。
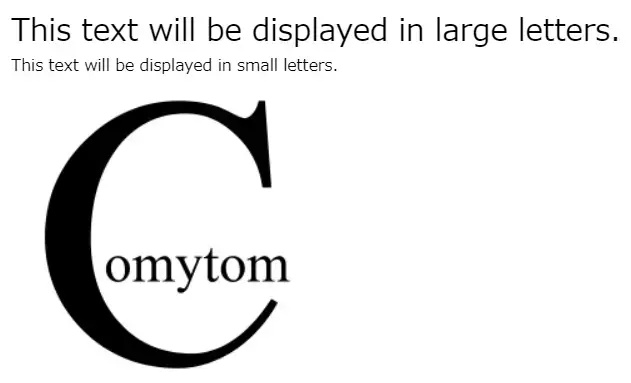
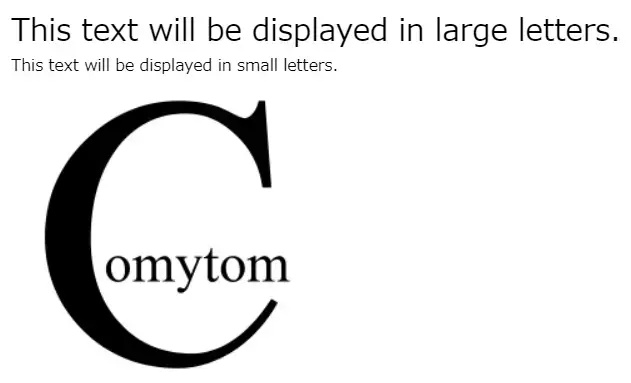
Vue Dynamic Componentsの使い方
Vue 3 Nuxt 3 Dynamic components are useful when you are unsure of what content you want to display or in what order. This article shows how to use dynamic components in Vue 3. The example uses Nuxt 3.
by JanitorSep 10, 2023
Dynamic Componentsの使い方の記事です。表示させたいコンテンツが、何なのか、またどのような順番でくるのか不明な時などに使うと便利です。
Dynamic Componentsの使い方の記事です。表示させたいコンテンツが、何なのか、またどのような順番でくるのか不明な時などに使うと便利です。
Jump Links
1. pagesフォルダにcomponentStudyフォルダを作成
2. componentを作成
3. 動作確認
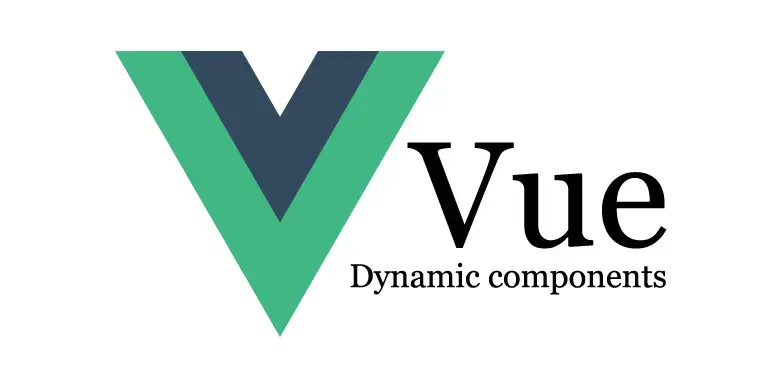
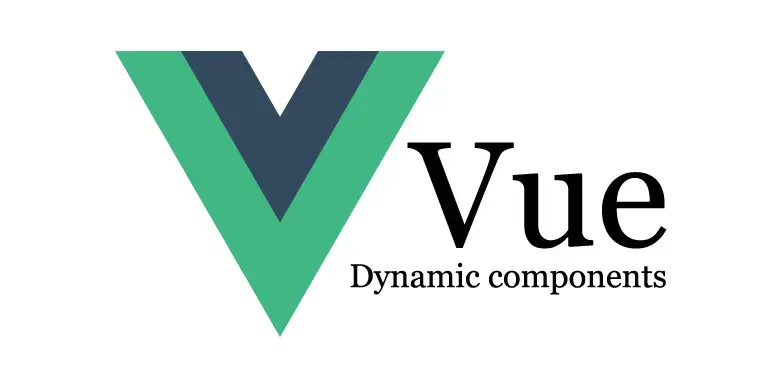
1. pagesフォルダにcomponentStudyフォルダを作成
1. pagesフォルダにcomponentStudyフォルダを作成
フォルダ名は何でも構いません。今回は「componentStudy」としています。直下にindex.vueを置きます。
フォルダ名は何でも構いません。今回は「componentStudy」としています。直下にindex.vueを置きます。
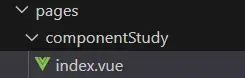
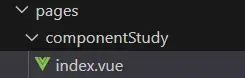
index.vueの内容は下記のようにして確認しています。やっていることは、表示データを準備し、その表示データのプロパティ「kind」によってvueを設定し、componentタグのis属性に設定したvueを読み込ませています。
index.vueの内容は下記のようにして確認しています。やっていることは、表示データを準備し、その表示データのプロパティ「kind」によってvueを設定し、componentタグのis属性に設定したvueを読み込ませています。
<script setup>
import fontSizeLargeVue from '@/components/fontSizeLarge.vue'
import fontSizeSmallVue from '@/components/fontSizeSmall.vue'
import imageVue from '@/components/image.vue'
// API等で表示データを取得
var contents = [
{ kind: "fontSizeLarge", arg: "This text will be displayed in large letters." },
{ kind: "fontSizeSmall", arg: "This text will be displayed in small letters." },
{ kind: "img", arg: "https://comytom.com/C250.webp" },
];
// どのvueを使うかを決定
for( var i = 0; i < contents.length; i++ ){
if( contents[ i ].kind == "fontSizeLarge" ) contents[ i ].vue = fontSizeLargeVue;
else if( contents[ i ].kind == "fontSizeSmall" ) contents[ i ].vue = fontSizeSmallVue;
else if( contents[ i ].kind == "img" ) contents[ i ].vue = imageVue;
}
</script>
<template>
<VContainer fluid class="h-100">
<component v-for="(content, i) in contents" :key="i" :is="{...content.vue}" :arg="content.arg"></component>
</VContainer>
</template>
<script setup>
import fontSizeLargeVue from '@/components/fontSizeLarge.vue'
import fontSizeSmallVue from '@/components/fontSizeSmall.vue'
import imageVue from '@/components/image.vue'
// API等で表示データを取得
var contents = [
{ kind: "fontSizeLarge", arg: "This text will be displayed in large letters." },
{ kind: "fontSizeSmall", arg: "This text will be displayed in small letters." },
{ kind: "img", arg: "https://comytom.com/C250.webp" },
];
// どのvueを使うかを決定
for( var i = 0; i < contents.length; i++ ){
if( contents[ i ].kind == "fontSizeLarge" ) contents[ i ].vue = fontSizeLargeVue;
else if( contents[ i ].kind == "fontSizeSmall" ) contents[ i ].vue = fontSizeSmallVue;
else if( contents[ i ].kind == "img" ) contents[ i ].vue = imageVue;
}
</script>
<template>
<VContainer fluid class="h-100">
<component v-for="(content, i) in contents" :key="i" :is="{...content.vue}" :arg="content.arg"></component>
</VContainer>
</template>
2. componentを作成
2. componentを作成
プロジェクトフォルダのcomponentsフォルダ(無い場合は作成)内に、上のスクリプトでimportしているコンポーネント3つ(fontSizeLarge.vue、fontSizeSmall.vue、image.vue)を作成します。
プロジェクトフォルダのcomponentsフォルダ(無い場合は作成)内に、上のスクリプトでimportしているコンポーネント3つ(fontSizeLarge.vue、fontSizeSmall.vue、image.vue)を作成します。
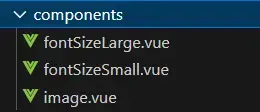
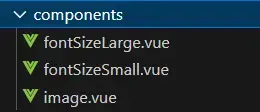
それぞれ下記のようにして、動的コンポーネントの動作を確認できます。
それぞれ下記のようにして、動的コンポーネントの動作を確認できます。
fontSizeLarge.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeLargeStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeLargeStyle{
font-size: 1.5rem;
}
</style>
fontSizeLarge.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeLargeStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeLargeStyle{
font-size: 1.5rem;
}
</style>
fontSizeSmall.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeSmallStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeSmallStyle{
font-size: 0.8rem;
}
</style>
fontSizeSmall.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<div
class="text-black fontSizeSmallStyle">
{{ arg }}
</div>
</template>
<style>
.fontSizeSmallStyle{
font-size: 0.8rem;
}
</style>
image.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<img :src="arg"
class="imageStyle">
</template>
<style>
.imageStyle{
width: 250px;
height: 250px;
}
</style>
image.vue
<script setup>
const props = defineProps( {
arg: String,
} );
</script>
<template>
<img :src="arg"
class="imageStyle">
</template>
<style>
.imageStyle{
width: 250px;
height: 250px;
}
</style>
参考にさせて頂いたウェブサイトURL
https://blog.logrocket.com/making-components-dynamic-vue-3/
参考にさせて頂いたウェブサイトURL
https://blog.logrocket.com/making-components-dynamic-vue-3/
3. 動作確認
3. 動作確認
yarn devしてcomponentStudyにアクセスすると下の画像のような表示になります。
yarn devしてcomponentStudyにアクセスすると下の画像のような表示になります。
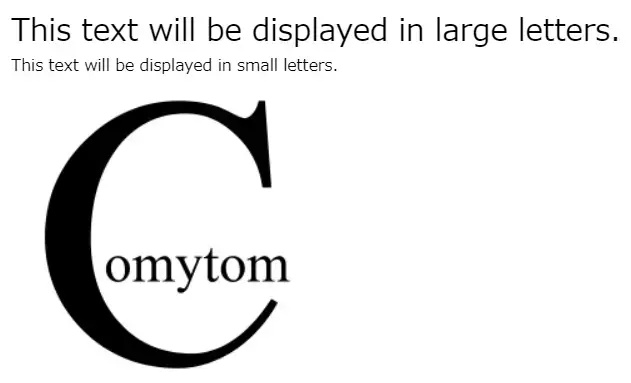
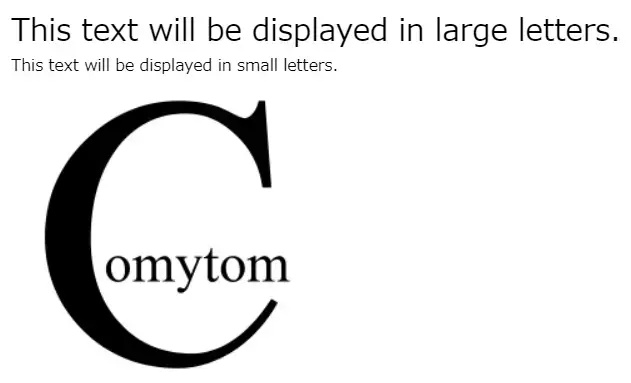