Script Labでセルのフォーマット
TosmmiOffice JavaScript API numberFormat HTML CSS Office JavaScript API This article provides a method to format numbers and dates entered in cells using the Office JavaScript API. It demonstrates how to format the number of decimal places and date formats by setting the format to a property called numberFormat. The accompanying HTML and CSS code allows users to enter input for number of decimal places and date format, and then executes the formatting functions using the Office JavaScript API.
by JanitorMar 07, 2024
Office JavaScript APIでセルに入力されている数値や日付のフォーマットを行う方法です。この記事では小数点以下の桁数のフォーマットの方法と、日付のフォーマットを例にしています。どちらもnumberFormatというプロパティに書式を設定することで実行できます。
Office JavaScript APIでセルに入力されている数値や日付のフォーマットを行う方法です。この記事では小数点以下の桁数のフォーマットの方法と、日付のフォーマットを例にしています。どちらもnumberFormatというプロパティに書式を設定することで実行できます。
Jump Links
1. 画面の例
2. Scriptを書く
3.HTMLとCSSを書く
3.実行
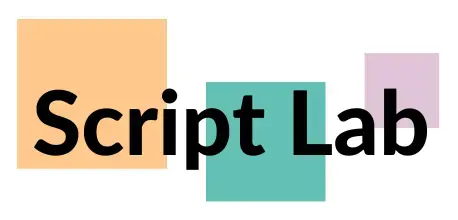
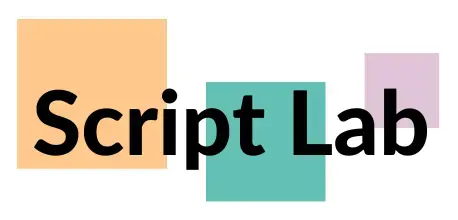
1. 画面の例
1. 画面の例
下の画像のようなUIにしています。オレンジの枠線内は、小数点桁数を入力するinputとその桁数でフォーマットを実行するボタンです。緑枠線内は、日付フォーマットを選択するラジオボタンと選択されたフォーマットを実行するボタンです。その下にある「Clear」ボタンはシート全体のフォーマットをクリアするボタンです。
下の画像のようなUIにしています。オレンジの枠線内は、小数点桁数を入力するinputとその桁数でフォーマットを実行するボタンです。緑枠線内は、日付フォーマットを選択するラジオボタンと選択されたフォーマットを実行するボタンです。その下にある「Clear」ボタンはシート全体のフォーマットをクリアするボタンです。
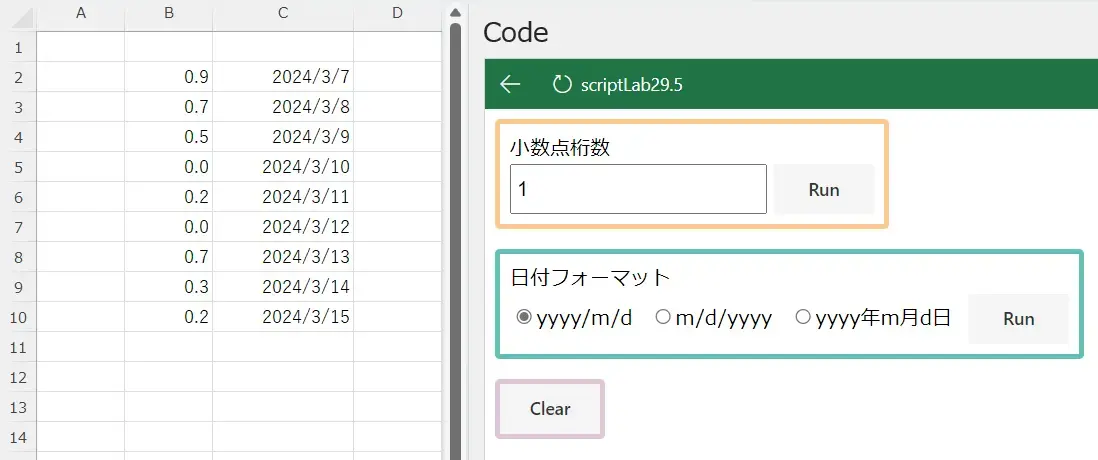
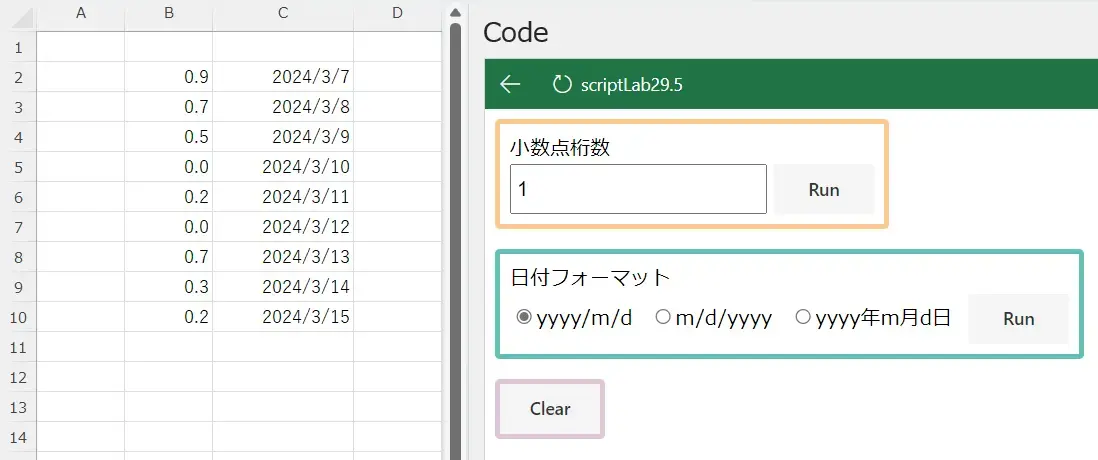
2. Scriptを書く
2. Scriptを書く
下のようなスクリプトを作成しました。
オレンジ枠線内の「Run」ボタンを押すと、「formatB」という関数が実行されます。「formatB」では、小数点桁数が入力されたinputを読み取り、その桁数に応じて書式を決めて、B列のnumberFormatプロパティにその書式を設定しています。
緑枠線内の「Run」ボタンを押すと「formatC」という関数が実行されま。「formatC」では、ラジオボタンのvalueに事前にフォーマット形式を設定しておき、ラジオボタンの選択値を読み取ったら、そのラジオボタンのvalueをC列のnumberFormatプロパティに設定しています。
下のようなスクリプトを作成しました。
オレンジ枠線内の「Run」ボタンを押すと、「formatB」という関数が実行されます。「formatB」では、小数点桁数が入力されたinputを読み取り、その桁数に応じて書式を決めて、B列のnumberFormatプロパティにその書式を設定しています。
緑枠線内の「Run」ボタンを押すと「formatC」という関数が実行されま。「formatC」では、ラジオボタンのvalueに事前にフォーマット形式を設定しておき、ラジオボタンの選択値を読み取ったら、そのラジオボタンのvalueをC列のnumberFormatプロパティに設定しています。
Script
$("#formatB").on("click", () => tryCatch(formatB));
$("#formatC").on("click", () => tryCatch(formatC));
$("#clear").on("click", () => tryCatch(clear));
async function formatB() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let bColumn = sheet
.getRange("B:B")
.getUsedRange()
.load();
await context.sync();
let formatStr = "0";
let numberOfDecimals = Number(document.getElementById("numberOfDecimals").value);
if (numberOfDecimals < 0) numberOfDecimals = 0;
else {
if (numberOfDecimals != 0) formatStr = formatStr + ".";
for (let i = 0; i < numberOfDecimals; i++) {
formatStr = formatStr + "0";
}
}
let formats = [];
for (let i = 0; i < bColumn.values.length; i++) {
formats.push([formatStr]);
}
bColumn.numberFormat = formats;
await context.sync();
});
}
async function formatC() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let cColumn = sheet
.getRange("C:C")
.getUsedRange()
.load();
await context.sync();
let formatStr = "yyyy/m/d";
let dateFormatSelects = document.getElementsByClassName("dateFormat");
for (let i = 0; i < dateFormatSelects.length; i++) {
if (dateFormatSelects[i].checked) {
formatStr = dateFormatSelects[i].value;
break;
}
}
let formats = [];
for (let i = 0; i < cColumn.values.length; i++) {
formats.push([formatStr]);
}
cColumn.numberFormat = formats;
await context.sync();
});
}
async function clear() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let usedRange = sheet.getUsedRange().load();
await context.sync();
usedRange.clear(Excel.ClearApplyTo.formats);
});
}
/** Default helper for invoking an action and handling errors. */
async function tryCatch(callback) {
try {
await callback();
} catch (error) {
// Note: In a production add-in, you'd want to notify the user through your add-in's UI.
console.error(error);
}
}
Script
$("#formatB").on("click", () => tryCatch(formatB));
$("#formatC").on("click", () => tryCatch(formatC));
$("#clear").on("click", () => tryCatch(clear));
async function formatB() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let bColumn = sheet
.getRange("B:B")
.getUsedRange()
.load();
await context.sync();
let formatStr = "0";
let numberOfDecimals = Number(document.getElementById("numberOfDecimals").value);
if (numberOfDecimals < 0) numberOfDecimals = 0;
else {
if (numberOfDecimals != 0) formatStr = formatStr + ".";
for (let i = 0; i < numberOfDecimals; i++) {
formatStr = formatStr + "0";
}
}
let formats = [];
for (let i = 0; i < bColumn.values.length; i++) {
formats.push([formatStr]);
}
bColumn.numberFormat = formats;
await context.sync();
});
}
async function formatC() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let cColumn = sheet
.getRange("C:C")
.getUsedRange()
.load();
await context.sync();
let formatStr = "yyyy/m/d";
let dateFormatSelects = document.getElementsByClassName("dateFormat");
for (let i = 0; i < dateFormatSelects.length; i++) {
if (dateFormatSelects[i].checked) {
formatStr = dateFormatSelects[i].value;
break;
}
}
let formats = [];
for (let i = 0; i < cColumn.values.length; i++) {
formats.push([formatStr]);
}
cColumn.numberFormat = formats;
await context.sync();
});
}
async function clear() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let usedRange = sheet.getUsedRange().load();
await context.sync();
usedRange.clear(Excel.ClearApplyTo.formats);
});
}
/** Default helper for invoking an action and handling errors. */
async function tryCatch(callback) {
try {
await callback();
} catch (error) {
// Note: In a production add-in, you'd want to notify the user through your add-in's UI.
console.error(error);
}
}
3.HTMLとCSSを書く
3.HTMLとCSSを書く
HTMLは下記の通りです。小数点桁数を入力するinputと、日付フォーマットを選択するラジオボタン、Script内に書いた「formatB」「formatC」「clear」という関数を実行するボタンを配置しています。
HTMLは下記の通りです。小数点桁数を入力するinputと、日付フォーマットを選択するラジオボタン、Script内に書いた「formatB」「formatC」「clear」という関数を実行するボタンを配置しています。
HTML
<div class="borderOrange fitContentsWdith pa-2">
<p>小数点桁数</p>
<div class="h40px">
<input id="numberOfDecimals" type="number" class="inputDefault h-100" value="1"/>
<button id="formatB" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="mt-4 borderGreen fitContentsWdith pa-2">
<p>日付フォーマット</p>
<div class="h40px">
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy/m/d">yyyy/m/d
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="m/d/yyyy">m/d/yyyy
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy年m月d日">yyyy年m月d日
</span>
<button id="formatC" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="h40px mt-4 borderPurple fitContentsWdith">
<button id="clear" class="ms-Button h-100">
<span class="ms-Button-label">Clear</span>
</button>
</div>
HTML
<div class="borderOrange fitContentsWdith pa-2">
<p>小数点桁数</p>
<div class="h40px">
<input id="numberOfDecimals" type="number" class="inputDefault h-100" value="1"/>
<button id="formatB" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="mt-4 borderGreen fitContentsWdith pa-2">
<p>日付フォーマット</p>
<div class="h40px">
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy/m/d">yyyy/m/d
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="m/d/yyyy">m/d/yyyy
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy年m月d日">yyyy年m月d日
</span>
<button id="formatC" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="h40px mt-4 borderPurple fitContentsWdith">
<button id="clear" class="ms-Button h-100">
<span class="ms-Button-label">Clear</span>
</button>
</div>
CSSは下記の通りです。html内のelementの高さや幅、マージンやパディング、ボーダーの色や幅を決めるクラスを作成しています。
CSSは下記の通りです。html内のelementの高さや幅、マージンやパディング、ボーダーの色や幅を決めるクラスを作成しています。
section.samples {
margin-top: 20px;
}
section.samples .ms-Button, section.setup .ms-Button {
display: block;
margin-bottom: 5px;
margin-left: 20px;
min-width: 80px;
}
p{
margin: 0px;
}
.inputDefault{
padding: 4px;
font-size: 16px;
box-sizing: border-box;
}
.h40px{
height: 40px;
}
.h-100{
height: 100% !important;
}
.mt-4{
margin-top: 16px;
}
.mr-2{
margin-right: 8px;
}
.pa-2{
padding: 8px;
}
.borderOrange{
border: 4px solid #FFC88D;
border-radius: 4px;
}
.borderGreen{
border: 4px solid #63C0B6;
border-radius: 4px;
}
.borderPurple{
border: 4px solid #E0C4D9;
border-radius: 4px;
}
.fitContentsWdith{
width: fit-content;
}
section.samples {
margin-top: 20px;
}
section.samples .ms-Button, section.setup .ms-Button {
display: block;
margin-bottom: 5px;
margin-left: 20px;
min-width: 80px;
}
p{
margin: 0px;
}
.inputDefault{
padding: 4px;
font-size: 16px;
box-sizing: border-box;
}
.h40px{
height: 40px;
}
.h-100{
height: 100% !important;
}
.mt-4{
margin-top: 16px;
}
.mr-2{
margin-right: 8px;
}
.pa-2{
padding: 8px;
}
.borderOrange{
border: 4px solid #FFC88D;
border-radius: 4px;
}
.borderGreen{
border: 4px solid #63C0B6;
border-radius: 4px;
}
.borderPurple{
border: 4px solid #E0C4D9;
border-radius: 4px;
}
.fitContentsWdith{
width: fit-content;
}
3.実行
3.実行
B列の2行目から10行目まで、エクセルの関数「RAND()」で埋めています。C列の2行目から10行目までは、日付を入力しました。小数点桁数を「2」、日付フォーマットのラジオボタンで「yyyy年m月d日」を選択してそれぞれ「Run」ボタンを実行すると下の画像のようになります。
B列の2行目から10行目まで、エクセルの関数「RAND()」で埋めています。C列の2行目から10行目までは、日付を入力しました。小数点桁数を「2」、日付フォーマットのラジオボタンで「yyyy年m月d日」を選択してそれぞれ「Run」ボタンを実行すると下の画像のようになります。
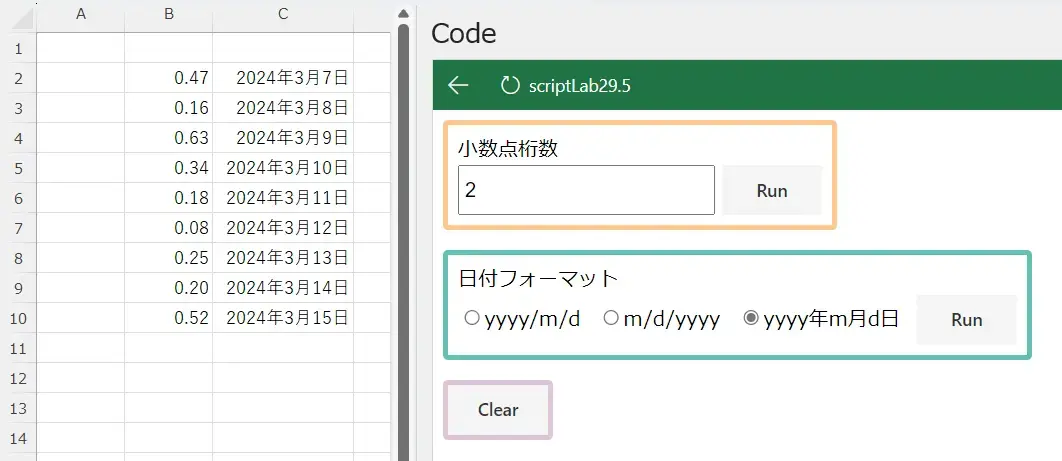
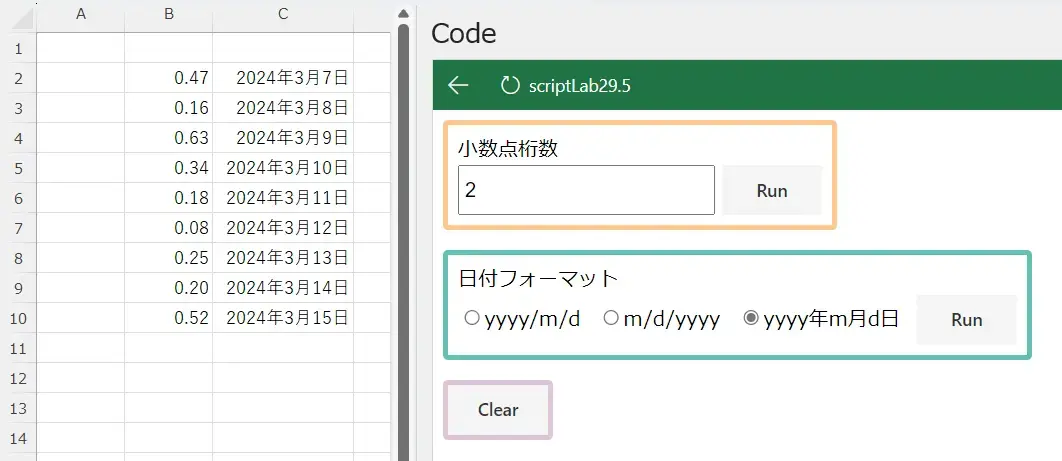
Script Labでセルのフォーマット
TosmmiOffice JavaScript API numberFormat HTML CSS Office JavaScript API This article provides a method to format numbers and dates entered in cells using the Office JavaScript API. It demonstrates how to format the number of decimal places and date formats by setting the format to a property called numberFormat. The accompanying HTML and CSS code allows users to enter input for number of decimal places and date format, and then executes the formatting functions using the Office JavaScript API.
by JanitorMar 07, 2024
Office JavaScript APIでセルに入力されている数値や日付のフォーマットを行う方法です。この記事では小数点以下の桁数のフォーマットの方法と、日付のフォーマットを例にしています。どちらもnumberFormatというプロパティに書式を設定することで実行できます。
Office JavaScript APIでセルに入力されている数値や日付のフォーマットを行う方法です。この記事では小数点以下の桁数のフォーマットの方法と、日付のフォーマットを例にしています。どちらもnumberFormatというプロパティに書式を設定することで実行できます。
Jump Links
1. 画面の例
2. Scriptを書く
3.HTMLとCSSを書く
3.実行
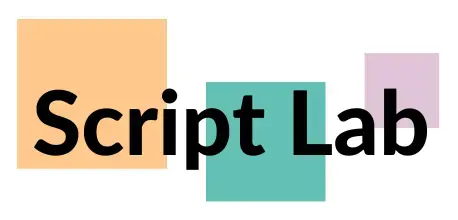
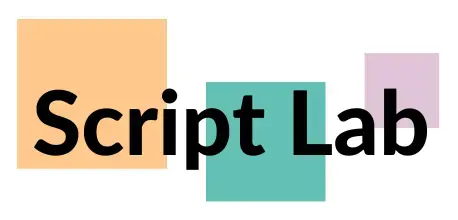
1. 画面の例
1. 画面の例
下の画像のようなUIにしています。オレンジの枠線内は、小数点桁数を入力するinputとその桁数でフォーマットを実行するボタンです。緑枠線内は、日付フォーマットを選択するラジオボタンと選択されたフォーマットを実行するボタンです。その下にある「Clear」ボタンはシート全体のフォーマットをクリアするボタンです。
下の画像のようなUIにしています。オレンジの枠線内は、小数点桁数を入力するinputとその桁数でフォーマットを実行するボタンです。緑枠線内は、日付フォーマットを選択するラジオボタンと選択されたフォーマットを実行するボタンです。その下にある「Clear」ボタンはシート全体のフォーマットをクリアするボタンです。
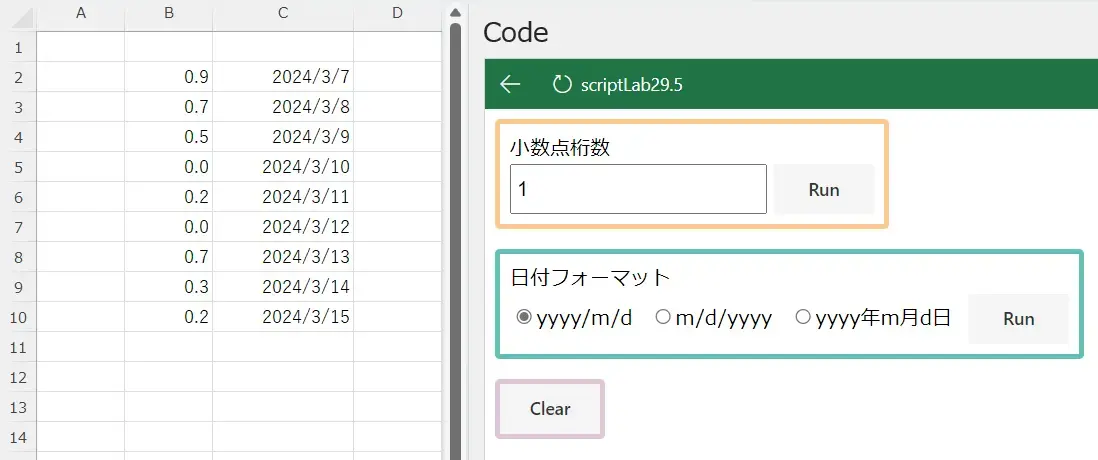
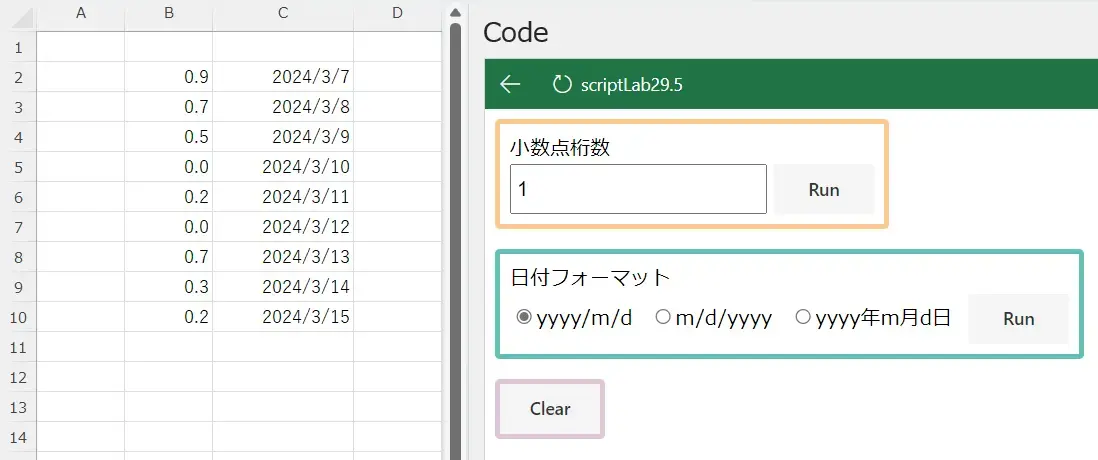
2. Scriptを書く
2. Scriptを書く
下のようなスクリプトを作成しました。
オレンジ枠線内の「Run」ボタンを押すと、「formatB」という関数が実行されます。「formatB」では、小数点桁数が入力されたinputを読み取り、その桁数に応じて書式を決めて、B列のnumberFormatプロパティにその書式を設定しています。
緑枠線内の「Run」ボタンを押すと「formatC」という関数が実行されま。「formatC」では、ラジオボタンのvalueに事前にフォーマット形式を設定しておき、ラジオボタンの選択値を読み取ったら、そのラジオボタンのvalueをC列のnumberFormatプロパティに設定しています。
下のようなスクリプトを作成しました。
オレンジ枠線内の「Run」ボタンを押すと、「formatB」という関数が実行されます。「formatB」では、小数点桁数が入力されたinputを読み取り、その桁数に応じて書式を決めて、B列のnumberFormatプロパティにその書式を設定しています。
緑枠線内の「Run」ボタンを押すと「formatC」という関数が実行されま。「formatC」では、ラジオボタンのvalueに事前にフォーマット形式を設定しておき、ラジオボタンの選択値を読み取ったら、そのラジオボタンのvalueをC列のnumberFormatプロパティに設定しています。
Script
$("#formatB").on("click", () => tryCatch(formatB));
$("#formatC").on("click", () => tryCatch(formatC));
$("#clear").on("click", () => tryCatch(clear));
async function formatB() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let bColumn = sheet
.getRange("B:B")
.getUsedRange()
.load();
await context.sync();
let formatStr = "0";
let numberOfDecimals = Number(document.getElementById("numberOfDecimals").value);
if (numberOfDecimals < 0) numberOfDecimals = 0;
else {
if (numberOfDecimals != 0) formatStr = formatStr + ".";
for (let i = 0; i < numberOfDecimals; i++) {
formatStr = formatStr + "0";
}
}
let formats = [];
for (let i = 0; i < bColumn.values.length; i++) {
formats.push([formatStr]);
}
bColumn.numberFormat = formats;
await context.sync();
});
}
async function formatC() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let cColumn = sheet
.getRange("C:C")
.getUsedRange()
.load();
await context.sync();
let formatStr = "yyyy/m/d";
let dateFormatSelects = document.getElementsByClassName("dateFormat");
for (let i = 0; i < dateFormatSelects.length; i++) {
if (dateFormatSelects[i].checked) {
formatStr = dateFormatSelects[i].value;
break;
}
}
let formats = [];
for (let i = 0; i < cColumn.values.length; i++) {
formats.push([formatStr]);
}
cColumn.numberFormat = formats;
await context.sync();
});
}
async function clear() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let usedRange = sheet.getUsedRange().load();
await context.sync();
usedRange.clear(Excel.ClearApplyTo.formats);
});
}
/** Default helper for invoking an action and handling errors. */
async function tryCatch(callback) {
try {
await callback();
} catch (error) {
// Note: In a production add-in, you'd want to notify the user through your add-in's UI.
console.error(error);
}
}
Script
$("#formatB").on("click", () => tryCatch(formatB));
$("#formatC").on("click", () => tryCatch(formatC));
$("#clear").on("click", () => tryCatch(clear));
async function formatB() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let bColumn = sheet
.getRange("B:B")
.getUsedRange()
.load();
await context.sync();
let formatStr = "0";
let numberOfDecimals = Number(document.getElementById("numberOfDecimals").value);
if (numberOfDecimals < 0) numberOfDecimals = 0;
else {
if (numberOfDecimals != 0) formatStr = formatStr + ".";
for (let i = 0; i < numberOfDecimals; i++) {
formatStr = formatStr + "0";
}
}
let formats = [];
for (let i = 0; i < bColumn.values.length; i++) {
formats.push([formatStr]);
}
bColumn.numberFormat = formats;
await context.sync();
});
}
async function formatC() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let cColumn = sheet
.getRange("C:C")
.getUsedRange()
.load();
await context.sync();
let formatStr = "yyyy/m/d";
let dateFormatSelects = document.getElementsByClassName("dateFormat");
for (let i = 0; i < dateFormatSelects.length; i++) {
if (dateFormatSelects[i].checked) {
formatStr = dateFormatSelects[i].value;
break;
}
}
let formats = [];
for (let i = 0; i < cColumn.values.length; i++) {
formats.push([formatStr]);
}
cColumn.numberFormat = formats;
await context.sync();
});
}
async function clear() {
await Excel.run(async (context) => {
const sheet = context.workbook.worksheets.getActiveWorksheet();
let usedRange = sheet.getUsedRange().load();
await context.sync();
usedRange.clear(Excel.ClearApplyTo.formats);
});
}
/** Default helper for invoking an action and handling errors. */
async function tryCatch(callback) {
try {
await callback();
} catch (error) {
// Note: In a production add-in, you'd want to notify the user through your add-in's UI.
console.error(error);
}
}
3.HTMLとCSSを書く
3.HTMLとCSSを書く
HTMLは下記の通りです。小数点桁数を入力するinputと、日付フォーマットを選択するラジオボタン、Script内に書いた「formatB」「formatC」「clear」という関数を実行するボタンを配置しています。
HTMLは下記の通りです。小数点桁数を入力するinputと、日付フォーマットを選択するラジオボタン、Script内に書いた「formatB」「formatC」「clear」という関数を実行するボタンを配置しています。
HTML
<div class="borderOrange fitContentsWdith pa-2">
<p>小数点桁数</p>
<div class="h40px">
<input id="numberOfDecimals" type="number" class="inputDefault h-100" value="1"/>
<button id="formatB" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="mt-4 borderGreen fitContentsWdith pa-2">
<p>日付フォーマット</p>
<div class="h40px">
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy/m/d">yyyy/m/d
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="m/d/yyyy">m/d/yyyy
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy年m月d日">yyyy年m月d日
</span>
<button id="formatC" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="h40px mt-4 borderPurple fitContentsWdith">
<button id="clear" class="ms-Button h-100">
<span class="ms-Button-label">Clear</span>
</button>
</div>
HTML
<div class="borderOrange fitContentsWdith pa-2">
<p>小数点桁数</p>
<div class="h40px">
<input id="numberOfDecimals" type="number" class="inputDefault h-100" value="1"/>
<button id="formatB" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="mt-4 borderGreen fitContentsWdith pa-2">
<p>日付フォーマット</p>
<div class="h40px">
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy/m/d">yyyy/m/d
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="m/d/yyyy">m/d/yyyy
</span>
<span class="mr-2">
<input type="radio" name="border" class="dateFormat" value="yyyy年m月d日">yyyy年m月d日
</span>
<button id="formatC" class="ms-Button h-100">
<span class="ms-Button-label">Run</span>
</button>
</div>
</div>
<div class="h40px mt-4 borderPurple fitContentsWdith">
<button id="clear" class="ms-Button h-100">
<span class="ms-Button-label">Clear</span>
</button>
</div>
CSSは下記の通りです。html内のelementの高さや幅、マージンやパディング、ボーダーの色や幅を決めるクラスを作成しています。
CSSは下記の通りです。html内のelementの高さや幅、マージンやパディング、ボーダーの色や幅を決めるクラスを作成しています。
section.samples {
margin-top: 20px;
}
section.samples .ms-Button, section.setup .ms-Button {
display: block;
margin-bottom: 5px;
margin-left: 20px;
min-width: 80px;
}
p{
margin: 0px;
}
.inputDefault{
padding: 4px;
font-size: 16px;
box-sizing: border-box;
}
.h40px{
height: 40px;
}
.h-100{
height: 100% !important;
}
.mt-4{
margin-top: 16px;
}
.mr-2{
margin-right: 8px;
}
.pa-2{
padding: 8px;
}
.borderOrange{
border: 4px solid #FFC88D;
border-radius: 4px;
}
.borderGreen{
border: 4px solid #63C0B6;
border-radius: 4px;
}
.borderPurple{
border: 4px solid #E0C4D9;
border-radius: 4px;
}
.fitContentsWdith{
width: fit-content;
}
section.samples {
margin-top: 20px;
}
section.samples .ms-Button, section.setup .ms-Button {
display: block;
margin-bottom: 5px;
margin-left: 20px;
min-width: 80px;
}
p{
margin: 0px;
}
.inputDefault{
padding: 4px;
font-size: 16px;
box-sizing: border-box;
}
.h40px{
height: 40px;
}
.h-100{
height: 100% !important;
}
.mt-4{
margin-top: 16px;
}
.mr-2{
margin-right: 8px;
}
.pa-2{
padding: 8px;
}
.borderOrange{
border: 4px solid #FFC88D;
border-radius: 4px;
}
.borderGreen{
border: 4px solid #63C0B6;
border-radius: 4px;
}
.borderPurple{
border: 4px solid #E0C4D9;
border-radius: 4px;
}
.fitContentsWdith{
width: fit-content;
}
3.実行
3.実行
B列の2行目から10行目まで、エクセルの関数「RAND()」で埋めています。C列の2行目から10行目までは、日付を入力しました。小数点桁数を「2」、日付フォーマットのラジオボタンで「yyyy年m月d日」を選択してそれぞれ「Run」ボタンを実行すると下の画像のようになります。
B列の2行目から10行目まで、エクセルの関数「RAND()」で埋めています。C列の2行目から10行目までは、日付を入力しました。小数点桁数を「2」、日付フォーマットのラジオボタンで「yyyy年m月d日」を選択してそれぞれ「Run」ボタンを実行すると下の画像のようになります。
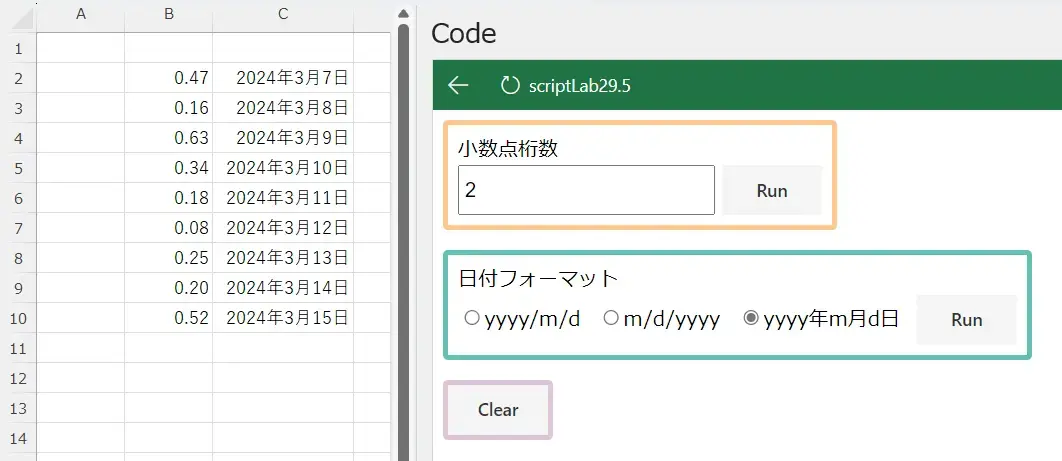
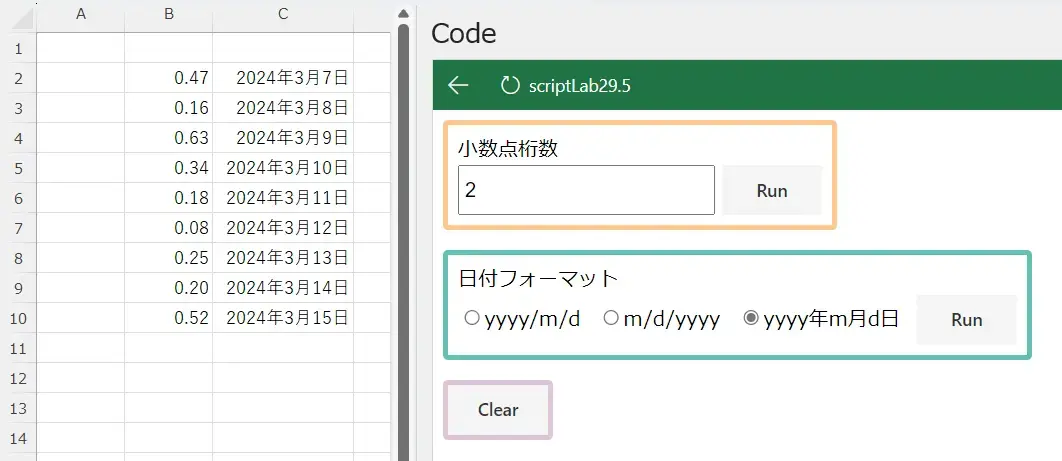